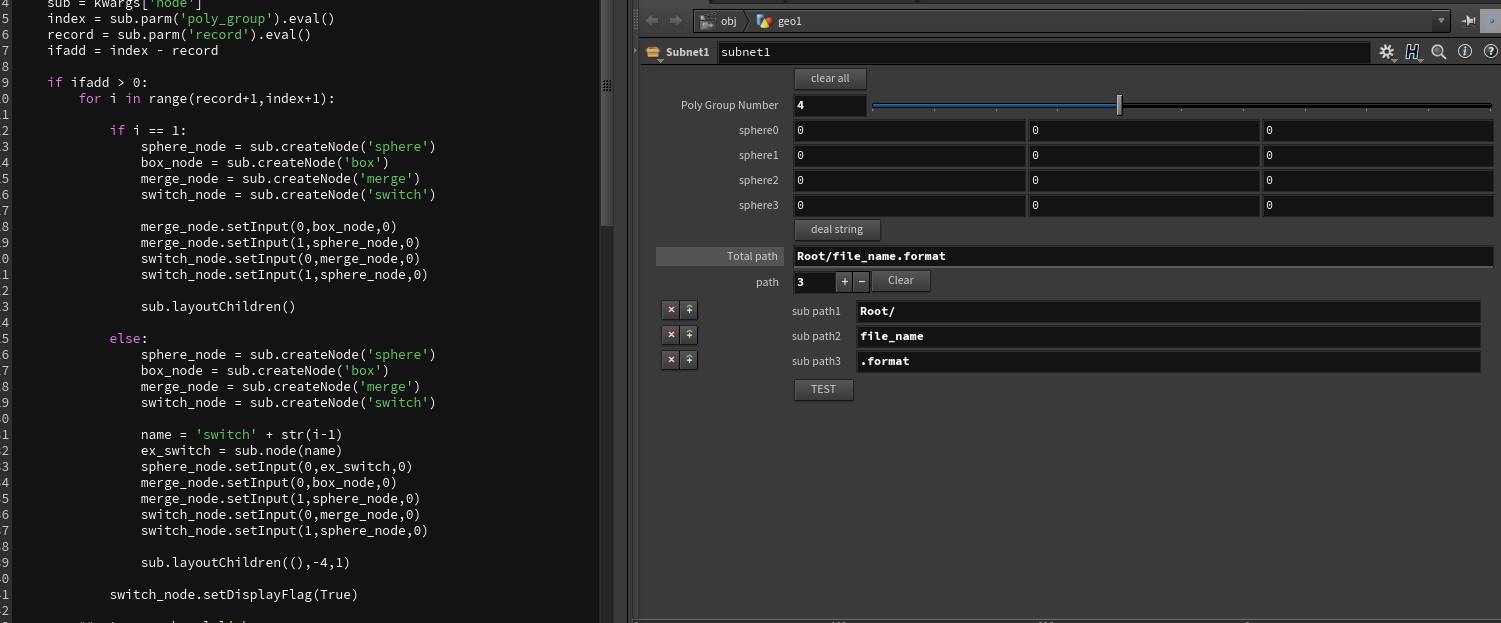
Houdini Node Management
This script generates a specified number of node combinations (which can be changed) within the subnetwork, and assigns a specified control link to each node combination.
def add_node(kwargs):
##Get node name and parm index
sub = kwargs['node']
index = sub.parm('poly_group').eval()
record = sub.parm('record').eval()
ifadd = index - record
if ifadd > 0:
for i in range(record+1,index+1):
if i == 1:
sphere_node = sub.createNode('sphere')
box_node = sub.createNode('box')
merge_node = sub.createNode('merge')
switch_node = sub.createNode('switch')
merge_node.setInput(0,box_node,0)
merge_node.setInput(1,sphere_node,0)
switch_node.setInput(0,merge_node,0)
switch_node.setInput(1,sphere_node,0)
sub.layoutChildren()
else:
sphere_node = sub.createNode('sphere')
box_node = sub.createNode('box')
merge_node = sub.createNode('merge')
switch_node = sub.createNode('switch')
name = 'switch' + str(i-1)
ex_switch = sub.node(name)
sphere_node.setInput(0,ex_switch,0)
merge_node.setInput(0,box_node,0)
merge_node.setInput(1,sphere_node,0)
switch_node.setInput(0,merge_node,0)
switch_node.setInput(1,sphere_node,0)
sub.layoutChildren((),-4,1)
switch_node.setDisplayFlag(True)
##set parm chanel link
pathx = 'ch("../sphere' + str(i-1) + 'x")'
pathy = 'ch("../sphere' + str(i-1) + 'y")'
pathz = 'ch("../sphere' + str(i-1) + 'z")'
sphere_node.parm('tx').setExpression(pathx,None,False)
sphere_node.parm('ty').setExpression(pathy,None,False)
sphere_node.parm('tz').setExpression(pathz,None,False)
else:
for i in range(index+1,record+1):
##delete nodes
switch = 'switch' + str(i)
merge = 'merge' + str(i)
box = 'box' + str(i)
sphere = 'sphere' + str(i)
sub.node(switch).destroy()
sub.node(merge).destroy()
sub.node(box).destroy()
sub.node(sphere).destroy()
sub.parm('record').set(index)
def clear(kwargs):
sub = kwargs['node']
sub.parm('poly_group').set(0)
sub.parm('record').set(0)
for node in sub.children():
node.destroy()
def add_path(kwargs):
node = kwargs['node']
index = node.parm('path').eval()
totalpath = ''
for i in range(1,index+1):
subpath = node.parm('sub_path'+str(i)).eval()
totalpath += subpath
node.parm('total_path').set(totalpath)
def add_matrial(kwargs):
node = kwargs['node'].parent
path = '/stage/materiallibrary1/principledshader'
path2 = '/stage/materiallibrary1/layermix1'
prin_node = hou.VopNetNode(path)
mix_node = hou.VopNetNode(path2)
mix_node.setFirstInput(prin_node,2)
prin_node.bypass(on)
express = '''a = 'aaaaaa'\nreturn a'''
def add(kwargs):
node = kwargs['node']
a = node.parent().createNode('groupcreate')
a.setExpressionLanguage(hou.exprLanguage.Python)
a.parm('basegroup').setExpression(express,None,False)